Unityにて格闘ゲームの練習になるようなアプリを作ってみた。
完成図↓
スマホ向けってことで、バーチャルパッドタイプ。
まずはコマンド用スクリプト↓
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using System.Collections.Generic;
using UnityStandardAssets.CrossPlatformInput;
public class Player2 : MonoBehaviour
{
private double LBtime;
private double Btime;
private double RBtime;
private double Ltime;
private double Rtime;
private double Crouchtime;
private Animator anim;
public float speed;
public List<string> command = new List<string>();
Rigidbody rb;
Image ray;
float x;
float y;
CapsuleCollider cc;
bool LB = true;
bool B = true;
bool RB = true;
bool L = true;
bool C = true;
bool R = true;
bool LF = true;
bool F = true;
bool RF = true;
// Use this for initialization
void Start()
{
anim = GetComponent<Animator>();
rb = GetComponent<Rigidbody>();
ray = GameObject.FindWithTag("Button").GetComponent<Image>();
cc = GameObject.FindWithTag("Down").GetComponent<CapsuleCollider>();
}
// Update is called once per frame
void Update()
{
if (LBtime >= 0.3f)
{
LBtime = 0.3f;
}
if (LBtime > 0)
{
LBtime -= Time.deltaTime;
}
if (Btime >= 0.4f)
{
Btime = 0.4f;
}
if (Btime > 0)
{
Btime -= Time.deltaTime;
}
if (RBtime >= 0.3f)
{
RBtime = 0.3f;
}
if (RBtime > 0)
{
RBtime -= Time.deltaTime;
}
if (Ltime >= 0.5f)
{
Ltime = 0.5f;
}
if (Ltime > 0)
{
Ltime -= Time.deltaTime;
}
if (Rtime >= 0.5f)
{
Rtime = 0.5f;
}
if (Rtime > 0)
{
Rtime -= Time.deltaTime;
}
if(Crouchtime >=0.8f)
{
Crouchtime = 0.8f;
}
if (anim.GetBool("Crouch") == false)
{
if (Crouchtime >= 0.4f)
{
Crouchtime -= Time.deltaTime * 2;
}
else if (Crouchtime <= 0.4f)
{
Crouchtime = 0;
}
}
x = CrossPlatformInputManager.GetAxis("Horizontal");
y = CrossPlatformInputManager.GetAxis("Vertical");
if (y < -0.5 && x < -0.5)
{
if (LB == true)
{
command.Add("1");
command.RemoveAt(0);
LB = false;
B = true;
RB = true;
L = true;
C = true;
R = true;
LF = true;
F = true;
RF = true;
}
LBtime += 0.4f;
Crouchtime += Time.deltaTime;
anim.SetBool("Run", false);
anim.SetBool("Crouch", true);
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
rb.rotation = Quaternion.Euler(0, 270, 0);
}
else if (y < -0.5 && x <= 0.5 && x >= -0.5)
{
if (B == true)
{
command.Add("2");
command.RemoveAt(0);
B = false;
LB = true;
RB = true;
L = true;
C = true;
R = true;
LF = true;
F = true;
RF = true;
}
Btime += 0.4f;
Crouchtime += Time.deltaTime;
anim.SetBool("Run", false);
anim.SetBool("Crouch", true);
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
}
else if (y < -0.5 && x > 0.5)
{
if (RB == true)
{
command.Add("3");
command.RemoveAt(0);
RB = false;
LB = true;
B = true;
L = true;
C = true;
R = true;
LF = true;
F = true;
RF = true;
}
RBtime += 0.4f;
Crouchtime += Time.deltaTime;
anim.SetBool("Run", false);
anim.SetBool("Crouch", true);
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
rb.rotation = Quaternion.Euler(0, 90, 0);
}
else if (y <= 0.5 && y >= -0.5 && x <= -0.5)
{
if (L == true)
{
command.Add("4");
command.RemoveAt(0);
L = false;
LB = true;
B = true;
RB = true;
C = true;
R = true;
LF = true;
F = true;
RF = true;
}
Ltime += 0.4f;
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
anim.SetBool("Crouch", false);
rb.rotation = Quaternion.Euler(0, 270, 0);
anim.SetBool("Run", true);
rb.velocity = new Vector3(-speed, 0, 0);
}
else if (y <= 0.5 && y >= -0.5 && x >= 0.5)
{
if (R == true)
{
command.Add("6");
command.RemoveAt(0);
R = false;
LB = true;
B = true;
RB = true;
L = true;
C = true;
LF = true;
F = true;
RF = true;
}
Rtime += 0.4f;
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
anim.SetBool("Crouch", false);
rb.rotation = Quaternion.Euler(0, 90, 0);
anim.SetBool("Run", true);
rb.velocity = new Vector3(speed, 0, 0);
}
else if (y > 0.5 && x <= -0.5)
{
if (LF == true)
{
command.Add("7");
command.RemoveAt(0);
LF = false;
LB = true;
B = true;
RB = true;
L = true;
C = true;
R = true;
F = true;
RF = true;
}
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
anim.SetBool("Run", false);
rb.rotation = Quaternion.Euler(0, 270, 0);
}
else if (y > 0.5 && x <= 0.5 && x >= -0.5)
{
if (F == true)
{
command.Add("8");
command.RemoveAt(0);
F = false;
LB = true;
B = true;
RB = true;
L = true;
C = true;
R = true;
LF = true;
RF = true;
}
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
anim.SetBool("Run", false);
}
else if (y > 0.5 && x > 0.5)
{
if (RF == true)
{
command.Add("9");
command.RemoveAt(0);
RF = false;
LB = true;
B = true;
RB = true;
L = true;
C = true;
R = true;
LF = true;
F = true;
}
if (anim.GetBool("Headspring") == true) return;
if (anim.GetBool("Rising_P") == true) return;
if (anim.GetBool("Jab") == true) return;
anim.SetBool("Run", false);
rb.rotation = Quaternion.Euler(0, 90, 0);
}
else
{
if (C == true)
{
anim.SetBool("Run", false);
anim.SetBool("Crouch", false);
C = false;
LB = true;
B = true;
RB = true;
L = true;
R = true;
LF = true;
F = true;
RF = true;
}
}
if (anim.GetBool("Crouch") == true)
{
cc.center = new Vector3(0, 0.2f);
}
else
{
cc.center = new Vector3(0, 0.7f, 0.05f);
}
}
public void P()
{
ray.raycastTarget = false;
if (anim.GetBool("Headspring") == true) return;
if (Rtime > 0 && Btime > 0 && RBtime > 0 && (command[2].Contains("6") || command[3].Contains("6") || command[4].Contains("6") || command[5].Contains("6")) && command[6].Contains("2") && command[7].Contains("3"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 90, 0);
rb.AddForce(1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (RBtime > 0 && Btime > 0 && command[5].Contains("3") && command[6].Contains("2") && command[7].Contains("3"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 90, 0);
rb.AddForce(1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Ltime > 0 && LBtime > 0 && Btime > 0 && RBtime > 0 && command[4].Contains("4") && command[5].Contains("1") && command[6].Contains("2") && command[7].Contains("3"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 90, 0);
rb.AddForce(1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Ltime > 0 && RBtime > 0 && Btime > 0 && LBtime > 0 && (command[1].Contains("4") || command[2].Contains("4") || command[3].Contains("4")) && (command[2].Contains("2") || command[3].Contains("2") || command[4].Contains("2")) && command[5].Contains("1") && command[6].Contains("2") && command[7].Contains("3"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 90, 0);
rb.AddForce(1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Ltime > 0 && Btime > 0 && LBtime > 0 && (command[2].Contains("4") || command[3].Contains("4") || command[4].Contains("4") || command[5].Contains("4")) && command[6].Contains("2") && command[7].Contains("1"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 270, 0);
rb.AddForce(-1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (LBtime > 0 && Btime > 0 && command[5].Contains("1") && command[6].Contains("2") && command[7].Contains("1"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 270, 0);
rb.AddForce(-1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Rtime > 0 && RBtime > 0 && Btime > 0 && LBtime > 0 && command[4].Contains("6") && command[5].Contains("3") && command[6].Contains("2") && command[7].Contains("1"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 270, 0);
rb.AddForce(-1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Rtime > 0 && RBtime > 0 && Btime > 0 && LBtime > 0 && (command[1].Contains("6") || command[2].Contains("6") || command[3].Contains("6")) && (command[2].Contains("2") || command[3].Contains("2") || command[4].Contains("2")) && command[5].Contains("3") && command[6].Contains("2") && command[7].Contains("1"))
{
anim.SetBool("Rising_P", true);
rb.rotation = Quaternion.Euler(0, 270, 0);
rb.AddForce(-1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Crouchtime >= 0.45f && command[7].Contains("7"))
{
anim.SetBool("Rising_P", true);
rb.velocity = new Vector3(0, 0, 0);
rb.rotation = Quaternion.Euler(0, 270, 0);
rb.AddForce(-1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (Crouchtime >= 0.45f && command[7].Contains("8"))
{
anim.SetBool("Rising_P", true);
if (rb.rotation == Quaternion.Euler(0, 90, 0))
{
rb.AddForce(1.3f, 8f, 0, ForceMode.VelocityChange);
}
else if (rb.rotation == Quaternion.Euler(0, 270, 0))
{
rb.AddForce(-1.3f, 8f, 0, ForceMode.VelocityChange);
}
}
else if (Crouchtime >= 0.45f && command[7].Contains("9"))
{
anim.SetBool("Rising_P", true);
rb.velocity = new Vector3(0, 0, 0);
rb.rotation = Quaternion.Euler(0, 90, 0);
rb.AddForce(1.3f, 8f, 0, ForceMode.VelocityChange);
}
else
{
anim.SetBool("Jab", true);
}
cc.center = new Vector3(0, 0.7f, 0.05f);
}
}
汚いスクリプトで申し訳ない…もっと良い方法があるんだろうけど…
バーチャルパッドの移動範囲でコマンド判定しています。
バーチャルパッドはスタンダードアセットと下記記事を参照しました。
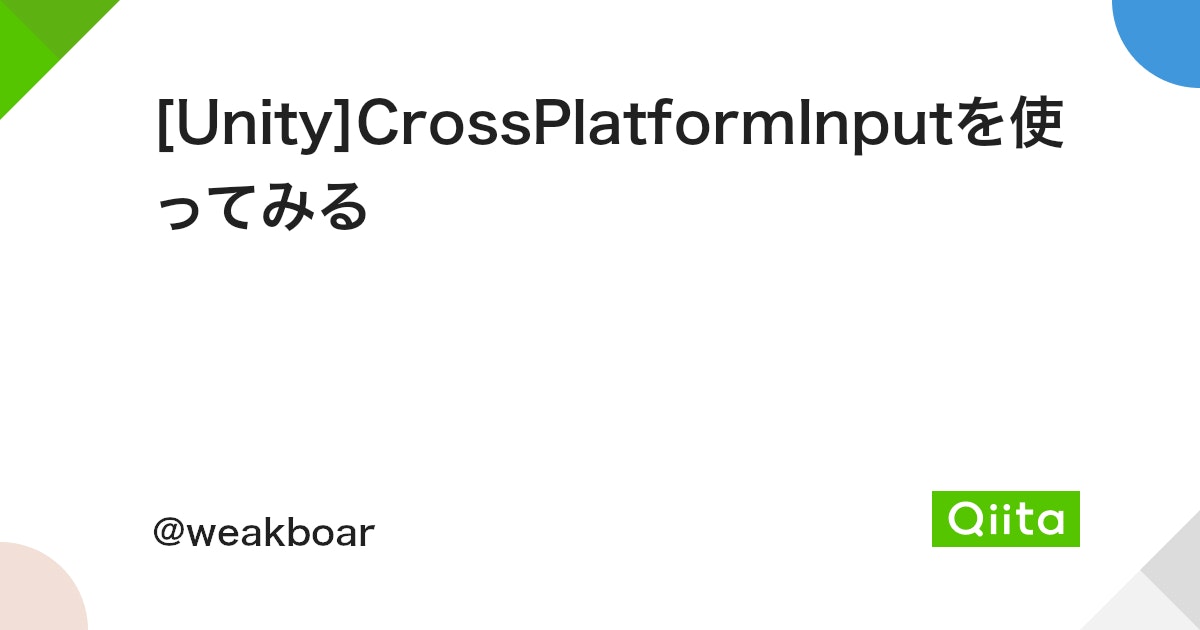
[Unity]CrossPlatformInputを使ってみる - Qiita
環境下記の環境を使用しています。Unity 5.4.1C#1.下準備からを選択しSwitch Platform[Asset…
バーチャルパッドのx軸が0.5以上、y軸が0.5~-0.5の範囲なら右に移動しますよ~。的な感じ。
これにコマンドと時間を付与する。
コマンドはListで管理。
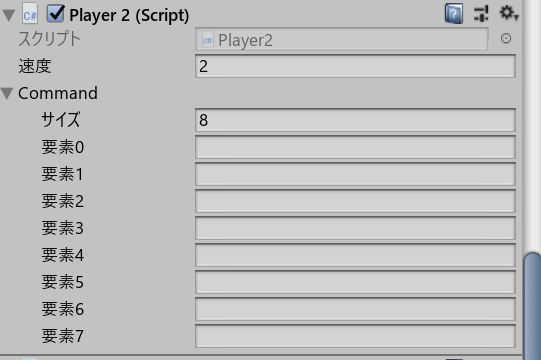
上記例でいけば、バーチャルパッドを右に入れてるので、”6”が要素8として追加され、要素0を削除して、最終的に要素7のところにコマンド”6”があるという感じ。
これに時間を持たせて、昇龍コマンド(623P)を入れるまでの受付時間のようにしています。
バーチャルパッドの仕様上、update内での処理しか思いつかなかった…。
コマンドを入れ続けると、listにコマンドが連続して入るのを防ぐため、bool使って、update内でコマンド入力を一度だけ入れる形を取りました。
これがInput系なら、ButtonDownとかで押したときだけコマンド判定とかにできるはず。受付時間はボタンが押されている間更新されるようにすれば良きかと。
んで、listの末尾3つが”6″,”2″,”3″+受付時間内でPを押せば技が出ますよー。それを満たしていないなら、普通のパンチですよー。という感じ。
ほかにコマンド判定の良い例があれば教えてほしい…。
Google playでダウンロードできるようになりました。↓
Not Found
キャラ、音源提供は下記
使用した音素材:OtoLogic(https://otologic.jp)
及び
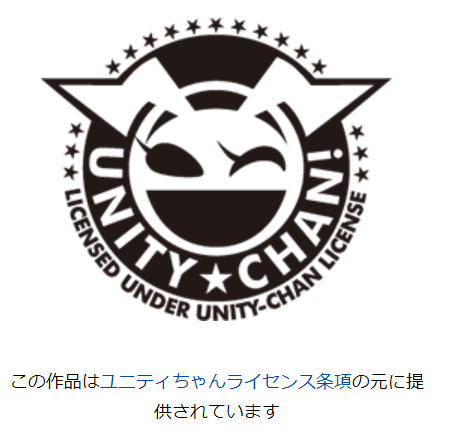
コメント
ソースが汚過ぎて、物を伝えるまでに至っていませんね。-_-b
コメントありがとうございます。
それは前コメントにも申しております。
趣味でゲームを作っており、コードの良し悪しはよく理解していないので、もしよろしければtommyさんの組むコードを拝見させていただけると幸いです。
これからの参考にさせていただきたいと思いますので、よろしくお願いします。
コメントありがとうございます。
備忘録程度の汚いスクリプトで、誰かに教える目的で組んだものではなかったので、コメントアウトなどは入れていません。実際に作ったゲームの操作するキャラのスクリプトをまるっと張り付けただけですので、冗長というより、すべてです。
ソースにコメントがなく冗長すぎで分かりづらいです。